Being a lazy programmer, I rarely write something twice. One instance of this was when I wanted to put items from a drop-down menu into a context menu but I didn’t want to copy them manually and attach to the same methods because I didn’t want to update the context menu every time that drop-down menu changed manually. So, I researched the web to see what the best way was to copy the drop-down menu items I wanted dynamically when my application loaded and still use the existing methods. As you can imagine, I found numerous ways of accomplishing the task. I picked the following method because it was the simplest. Obviously, it is not the only way but I thought it would be helpful to others if shared it.
Let’s say I have a drop-down menu (or any menu) that looks like the following:
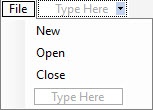
And I have a context menu that looks like the following:
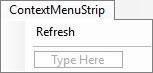
And I want to copy the items from the drop-down menu to the context menu after the separator when the application loads. I also do not want to rewrite the methods for the items in the drop-down menu.
The following foreach
loop in the main form load event will copy all dropdown menu items and add them to the context menu. The ExecuteMethod
method will determine which menu item was clicked and perform the click action for the original menu item (so you don’t have to write or link it again). You can also add conditions in the foreach
loop to limit which dropdown items are copied. But that depends on which property you wish to use for conditions.
private void MainForm_Load(object sender, EventArgs e)
{
// Loop through all dropdown menu items
foreach (ToolStripMenuItem myItem in fileToolStripMenuItem.DropDownItems) {
// Define a temporary dropdown item
ToolStripMenuItem tmpItem = new ToolStripMenuItem();
// Copy dropdown item name and append text Copy
tmpItem.Name = myItem.Name + "Copy";
// Copy dropdown item text
tmpItem.Text = myItem.Text;
// Copy downdown item image if necessary
tmpItem.Image = myItem.Image;
// Assign the click action to the ExecuteMethod method below
tmpItem.Click += ExecuteMethod;
// Add the copy menu item to the context menu
contextMenuStrip1.Items.Add(tmpItem);
}
}
private void ExecuteMethod(object sender, EventArgs e)
{
// When a context menu item is clicked, retrieve its name removing the appended text
string MenuItem = ((ToolStripMenuItem)sender).Name.Replace("Copy", "");
// Use the retrieved name to get the menu item from the original menu
ToolStripItem ClickedMenu = fileToolStripMenuItem.DropDownItems[MenuItem];
if (ClickedMenu.Enabled == true) {
// If the menu is enabled then perform the click action
ClickedMenu.PerformClick();
}
}
Code language: C# (cs)